Learning JavaScript can make web development much easier and more intuitive. In this tutorial, we’ll focus on how to use the focus and keyup event handlers to execute the confirmpasswordevent on line 51 on app.js. We’ll also cover how these event handlers work and how they can benefit your development.
Introduction to focus and keyup event handlers
The focus and keyup event handlers are commonly used in JavaScript for form validation and user input handling. The focus event handler is triggered when an HTML element, like an input field or button, gains focus. The keyup event handler is triggered when a key on the keyboard is released while the focus is on an element.
Once you have a basic understanding of these two event handlers, you can use them to execute more complex functions, like executing the confirmpasswordevent on line 51 of app.js. To do this, you first need to identify the password input field and add a focus event listener to it. Next, you need to add a keyup event listener to the password confirmation input field to execute the confirmpasswordevent function.
By using focus and keyup event handlers, you can create more dynamic and interactive user experiences on your website or web application.
On line 51 on app.js, use the focus and keyup event handlers to execute the confirmpasswordevent
The “confirmpassword” event is an important feature in JavaScript that is used to confirm whether the values entered in two password fields are identical or not.
Here’s how to use the focus and keyup event handlers to execute the confirmpassword event in your JavaScript code:
On line 51 of app.js, add the following code: |
const confirmPassword = document.getElementById(‘confirm-password’);
confirmPassword.addEventListener(‘focus’, function(){ confirmPassword.addEventListener(‘keyup’, function(){ const password = document.getElementById(‘password’).value; const confirmPasswordValue = confirmPassword.value; if (password != confirmPasswordValue){ confirmPassword.setCustomValidity(‘Passwords do not match’); } else { confirmPassword.setCustomValidity(”); } }); }); |
This code uses the focus and keyup event handlers to compare the values entered in the “password” and “confirm-password” fields. If the values are not identical, an error message will appear prompting the user to re-enter their password.
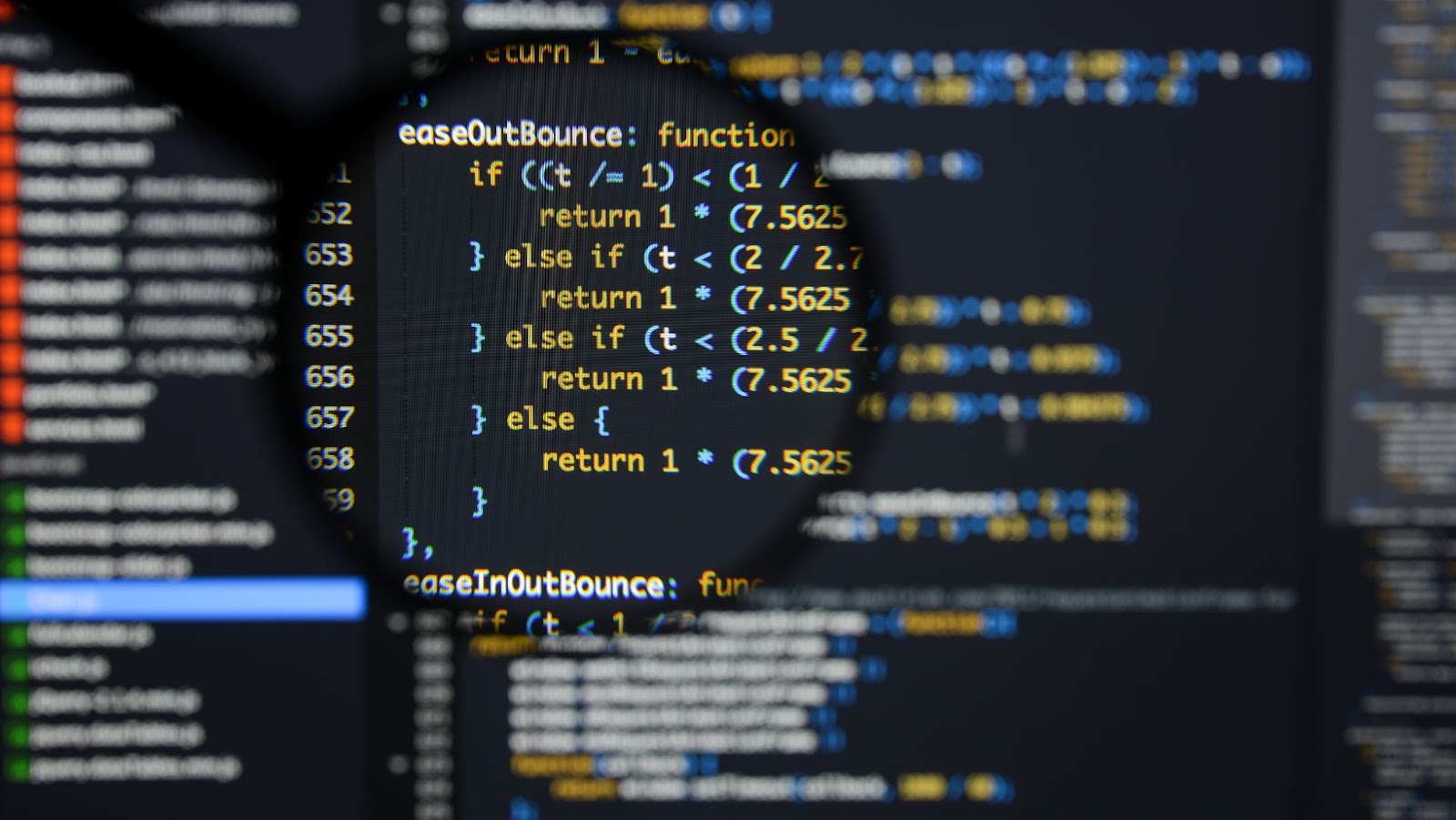
A href=’http://goo.gl/gyrz4b’ target=’_blank’>http://goo.gl/gyrz4b</a>
To implement the confirmpassword event on line 51 of app.js, you can use the focus and keyup event handlers in JavaScript. These event handlers will execute the confirmpassword function when the user focuses on the password field and types in the matching confirmation password respectively.
Here’s an example code snippet to get you started:
const passwordField = document.getElementById(“password”); |
const confirmPasswordField = document.getElementById(“confirm-password”); |
confirmPasswordField.addEventListener(“focus”, () => { |
// User focuses on the confirm password field |
confirmpassword(); |
}); |
confirmPasswordField.addEventListener(“keyup”, () => { |
// User types in the confirm password field |
confirmpassword(); |
}); |
function confirmpassword() { |
// Implement your logic to compare the passwords and display an error message if they don’t match |
} |
With this implementation, users will receive real-time feedback on whether their confirmation password matches the original password, ensuring data accuracy and preventing errors.
Working with Keyboard Events in JavaScript
In JavaScript, keyboard events are one of the most convenient ways to manage user input and interactivity on your webpages. a href=’http://goo.gl/gyrz4b’ target=’_blank’>http://goo.gl/gyrz4b</a> allow developers to access keyboard related activity such as the press and release of a key or combination of keys.
This tutorial will go through working with keyboard events in JavaScript, using code examples to illustrate the concepts. We will go over how to use the focus and keyup event handlers to execute the confirmpasswordevent on line 51 on app.js.
Different types of keyboard events
JavaScript provides different types of keyboard events that allow developers to add interactivity to their web applications. Some of the commonly used keyboard events are:
Event | Description |
Keydown event | This event occurs when a key is pressed down. |
Keypress event | This event occurs when a key is pressed down and produces a character value. |
Keyup event | This event occurs when a key is released. |
These events can be used to trigger different functions or behaviours in a web application. For example, by using the focus and keyup event handlers in JavaScript, you can execute the confirmpasswordevent on line 51 in app.js. This allows you to validate the user’s password in real-time as they type, providing a better user experience.
Pro Tip: Make sure to test your keyboard event functionality thoroughly across different devices and browsers to ensure maximum compatibility.
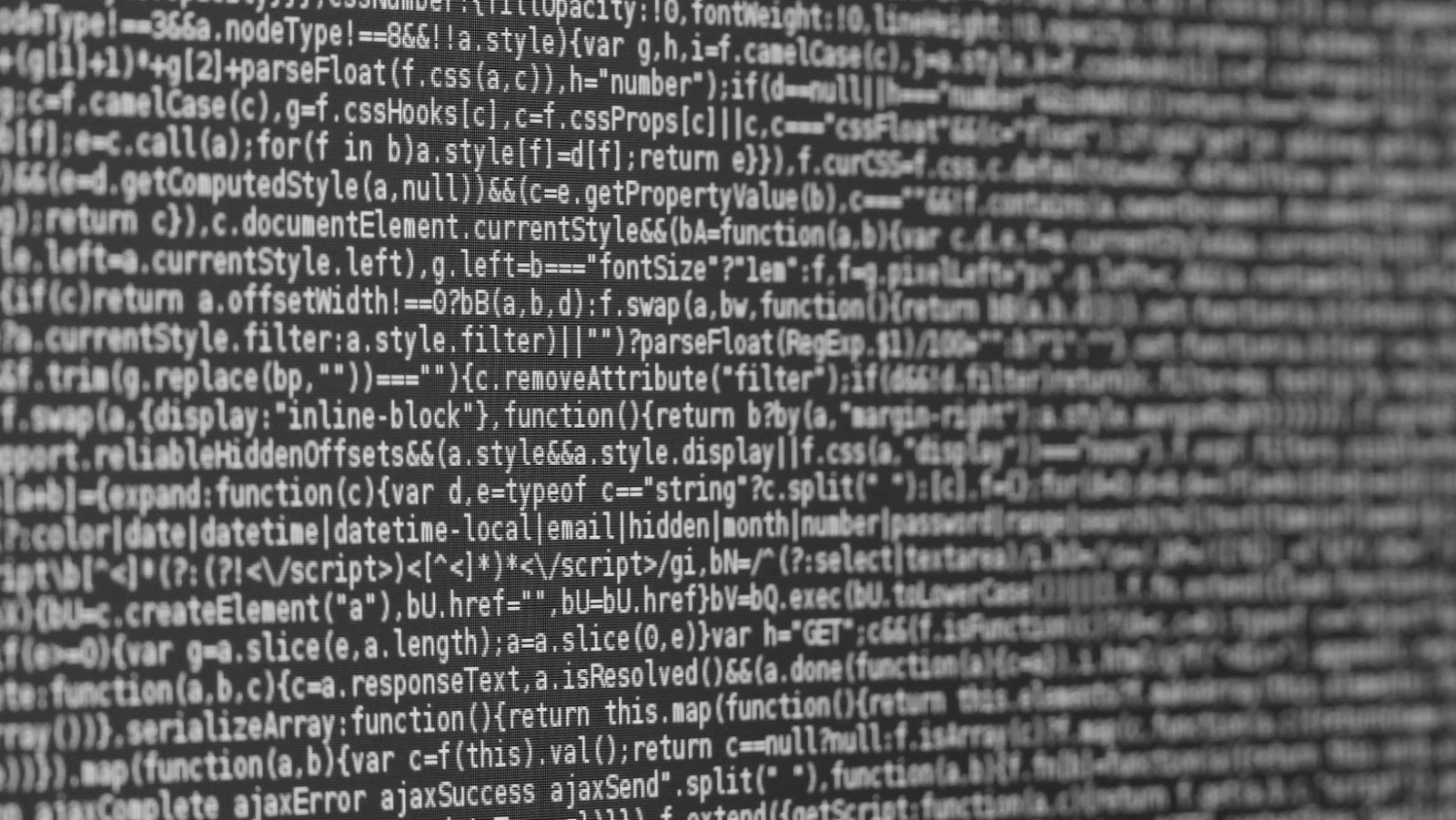
Examples of keyboard event handling in JavaScript
When it comes to building interactive web applications, handling keyboard events in JavaScript can be a vital aspect. Here are a few examples of keyboard event handling in JavaScript-
1. Keydown event: | This event is fired when a key is pressed down. You can use this event to respond to specific key presses, like arrow keys or the spacebar. |
2. Keyup event: | This event is fired when a key is released after being pressed. You can use this event to receive input from the user, like in a search box. |
3. Keypress event: | This event is triggered when a key is pressed down, and it produces a character value. This event is useful when working with input fields that require textual input. |
Here’s an example of keyboard event handling in JavaScript: On line 51 in app.js, you can use the “focus” and “keyup” event handlers to execute the “confirmpasswordevent” function when a user presses a key in a password confirm field.
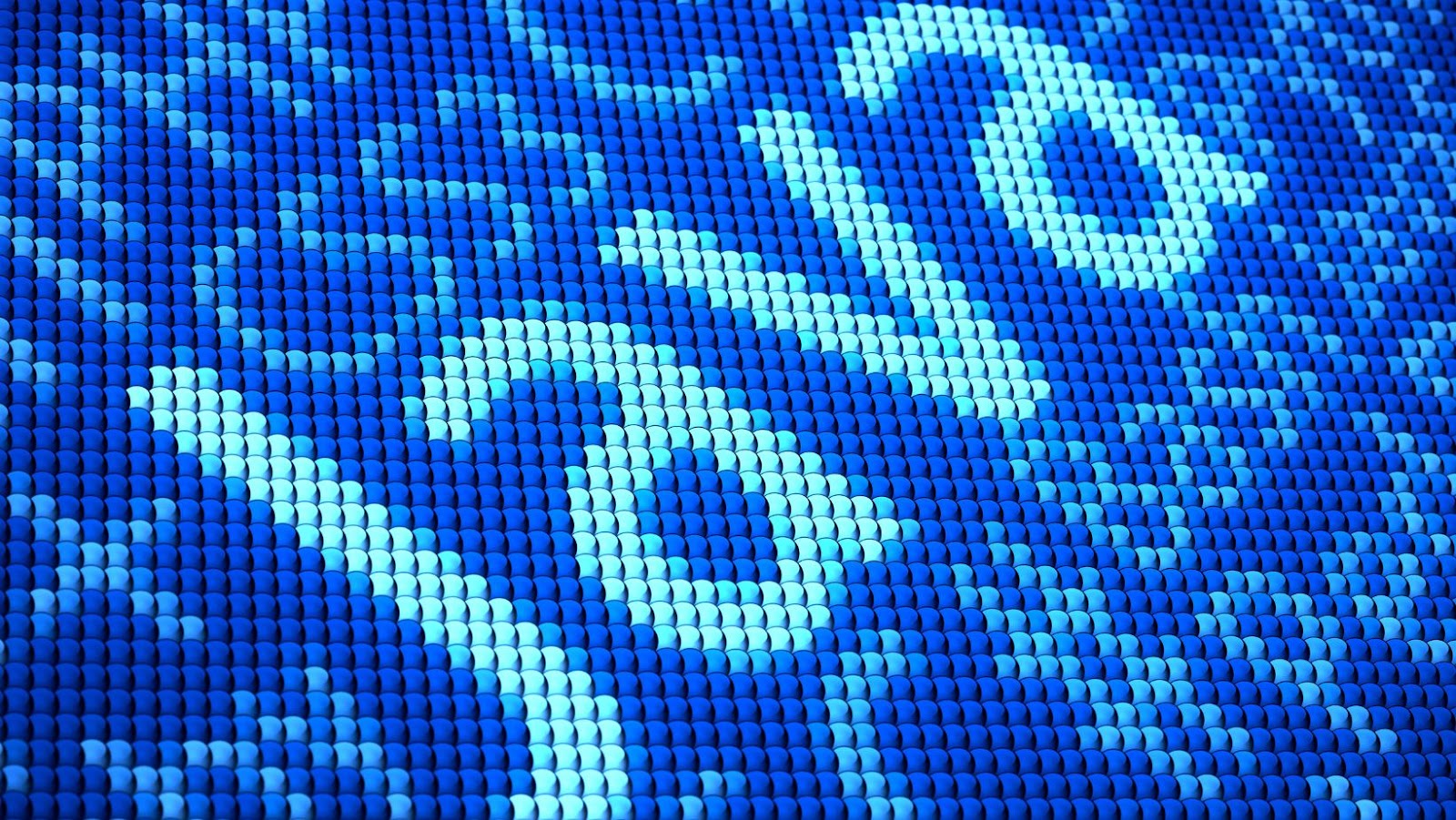
Best practices for using keyboard event handlers in JavaScript
When using keyboard event handlers in JavaScript, it is important to follow some best practices to ensure smooth and efficient execution of your code. Here are some tips to keep in mind:
- Avoid using key event handlers for non-text input elements like buttons and links.
- Use event delegation to handle events on multiple elements efficiently.
- Use the `event.key` property instead of `event.keyCode` for improved readability and cross-browser support.
- Consider using throttling or debouncing techniques to handle repeated or frequent key events.
To execute the `confirmpasswordevent` using the `focus` and `keyup` event handlers on line 51 in `app.js`, you can use the following code:
const passwordInput = document.getElementById(‘password’);
const confirmPasswordInput = document.getElementById(‘confirm-password’); passwordInput.addEventListener(‘focus’, () => { confirmPasswordInput.addEventListener(‘keyup’, confirmpasswordevent); }); |